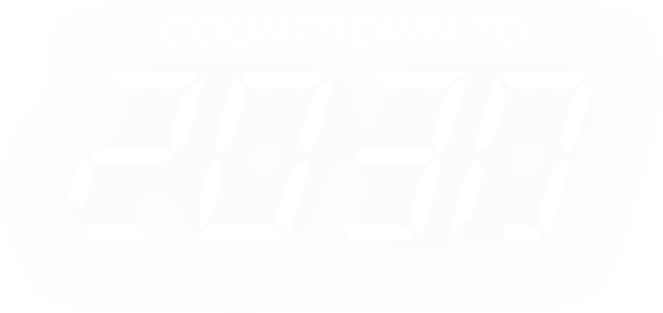
看到未来的潜力,不是过去的计划
加速收入增长
Excel将数据转换为市场机会
正确的产品,合适的人,正确的地方,正确的时间。Anaplan转变为差异化的优势,以推动销售业绩,获得市场份额,提高客户满意度。
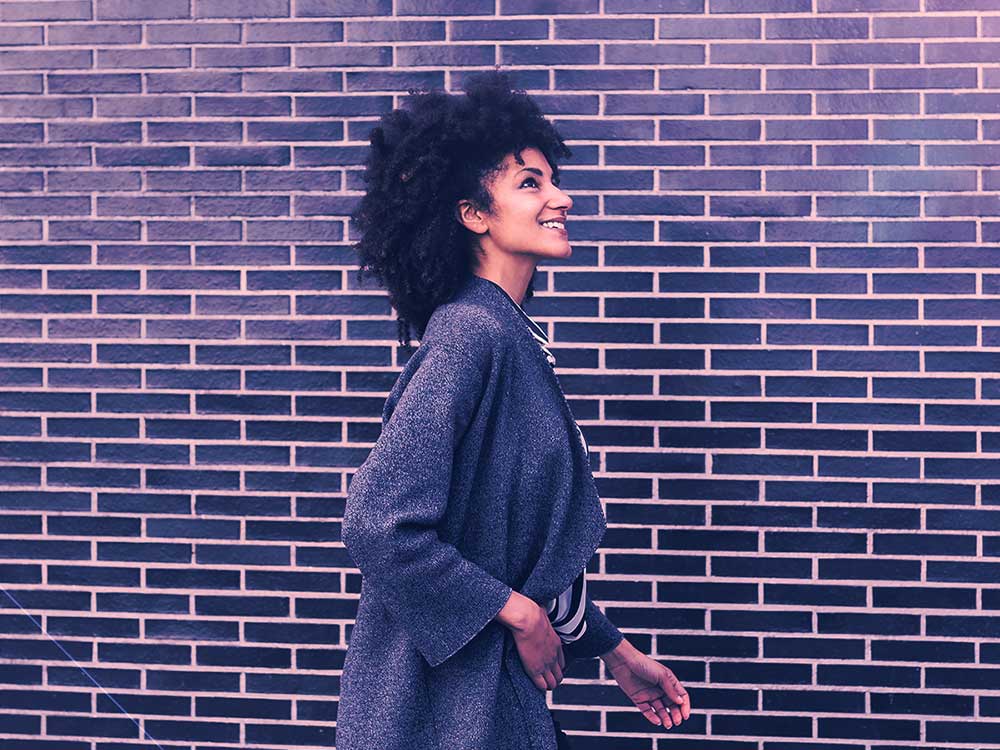
在下一个正常茁壮成长
亚搏彩票手机版免费下载在不断变化的环境中取得成功
常规转弯不好了进入A-HA.,克服你现在看到的挑战和你永远无法看到的人。Anaplan帮助您茁壮成长 - 不仅仅是生存 - 随着下一个正常的发展。
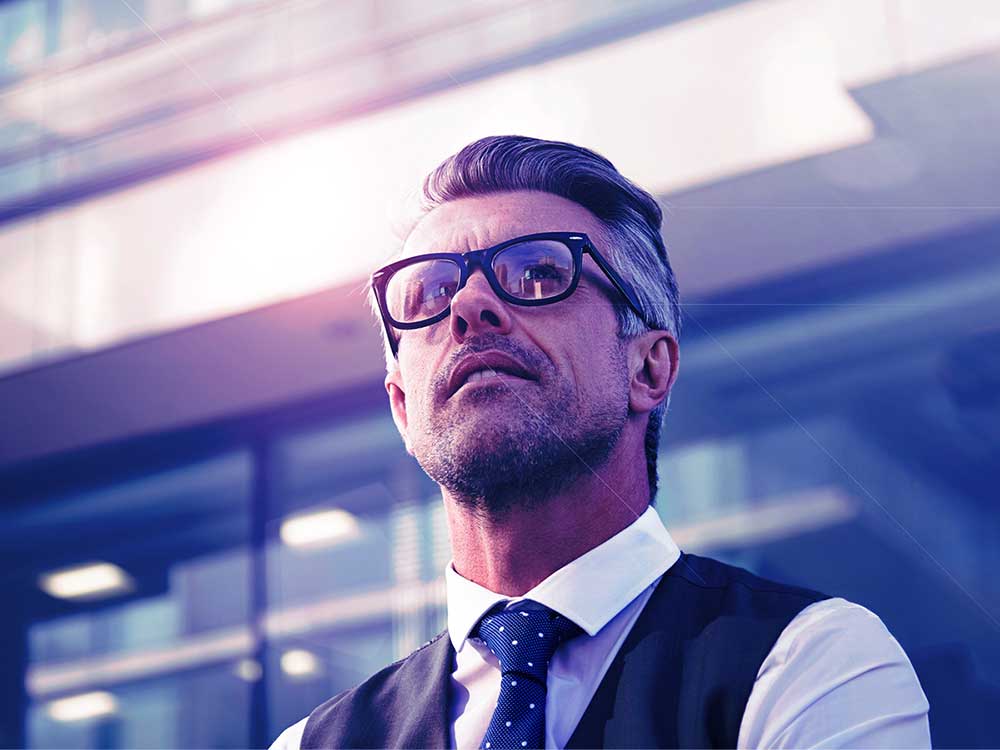
实现了未开发的潜力
集体突破增长的辉煌
赋予你的人民创造成功。亚搏彩票手机版免费下载ANAPLAN使信息随时可用,轻松分享,并对您的企业团队共享和强化自适应。
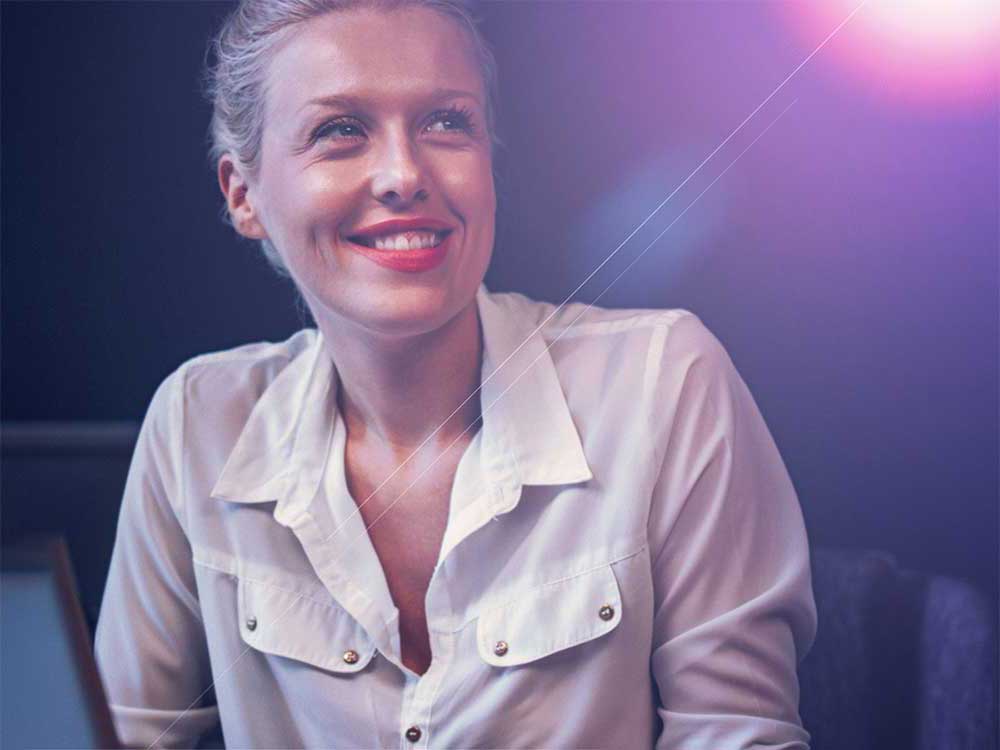
使用灵活性和弹性协调性能
在动态,不断变化和快速移动的景观中,
Anaplan将您的策略连接到您的结果并推动与单一真理来源的问责制
我们为尊重的行业分析师承认我们的开拓精神和我们全面发行的力量
世界各地的领先公司在世界各地选择Anaplan
领先的咨询公司将客户与Anaplan授权
今天开始使用Anaplan
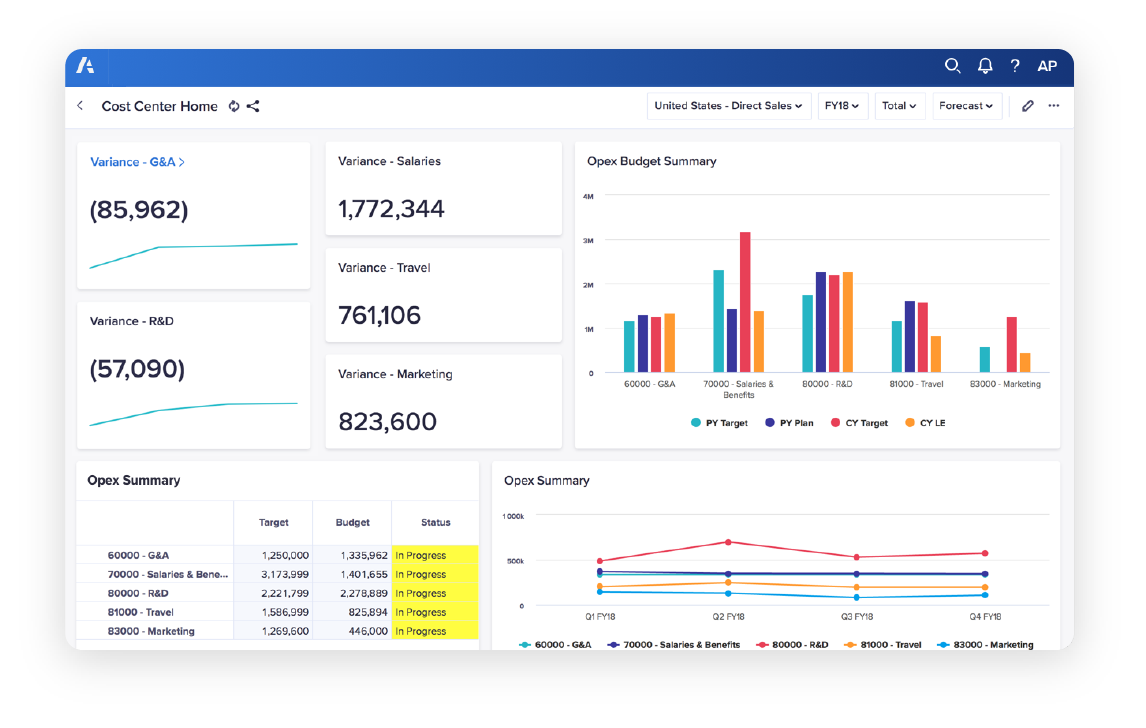